07 Apr 2018
This post explains how to expose your services hosted in your private data center to AWS accounts over AWS. I assume that you already have some understanding about Amazon Web Services and how things work in the world of AWS Cloud Services.
To begin with let’s first understand what is the new Private Link service launched by AWS in re:Invent 2017.
In re:Invent 2017, AWS announced a new service called Private Link. According to the announcement, “AWS PrivateLink is the newest generation of VPC Endpoints which is designed for customers to access AWS services in a highly available and scalable manner, while keeping all the traffic within the AWS network”. What this means is, you can create your own VPC endpoint in your AWS account and access services which are hosted in other AWS accounts. This service can be further extended and used to access services via endpoints hosted in your private data center.
In this blog post, I am going to explain how we can expose our service not hosted in AWS, but hosted in your private data center, to other accounts on AWS. So let’s begin.
AWS Direct Connect
AWS Direct Connect is one of the services provided by AWS which enables you to establish a dedicated network connection from your data center to AWS. This service is nothing but a VPN connection from your AWS account to your data center. You need to establish this because this is how your AWS account is going to talk to your service hosted in private data center.
Architecture
31 Aug 2017
Many a times Dev-Ops folks love to have a command-line application rather than going to the browser and doing things. And yes, why not. Command-line applications are a lot faster than the browser and can be scripted eaisly.
In this post I am wrting about how to build a command-line application in Python.
When I say command-line application one thing that urgently strikes to my mind is I have to build something like “ping” having various options like ‘-a’,’-v’ and also having a pretty ‘usage’.
Hence inorder to build such type of application you have to think about parsing arguments from command-line.
There are various tools our there which makes it easy to build a command-line application. Some of the examples are:
- Click
- Clint
- docopt
- Cliff
I started off by using Click. Click provide you with good api’s in order to create nested commands for your application. It also has its own way to handle user credentials and printing the usage string. Its pretty neat if you are looking to build a commmand line appliction which will be dependent upon python. But in my case I had to build an application which shall be independent of the fact that whether the user has python installed on his/her machine or no. In order to achieve this the best option left with me was to use something which comes with python installation itself. Hence I decided to move with argparse. arparse is not liked much by the python community because it is more code to write when compared to the above modules like Click or Clint. But it also has good documentation in place and once you get familiar with its functionality you start liking it.
My aim was to build a command line application with nested command which will do different things for the user. To give you an example it should look something like this:
$myCommandLineApplication doSomething -withThis String -alsoWithThis String
Now, if you observe, we cant just use Parser.ArgumentParser() and parse the strings given as command line arguments. We need something like subparser in order to tell the command line application that doSomething is supposed to parse the strings and not myCommandLineApplication. And just as we want argparse has a function called subparser which can be used in order to achieve the above goal.
07 Jul 2017
“Log in with Facebook”, “Log in with Google”. I think these are the two buttons which really makes us happy whenever we see them on any application we newly install or web application we browse. The reason being, we don’t have to go through the long process of creating an account for that application OR remembering the username/password for that particular application. So how does this “Log in with Google” thing works?
The short answer is they have implemented something called as Single-Sign On (SSO) solution. And in this blog post, I am writing about OAuth and SAML, the two specifications used in order to achieve single-sign on user experience.
SAML 2.0 Basics
Security Assertion Markup Language 2.0 is a version of SAML standard for exchanging authentication and authorization data between security domains. SAML 2.0 is an XML-based protocol that uses security based tokens containing assertions to pass information about the user between the web server you are trying to access information on and the service provider. In SAML 2.0 specification there are three main actors:
- Service Provider (Resource Server): This is the web server you are trying to access information on.
- Client: Your web browser
- Identity Provider (Authorization Server): This is the server that owns the user credentials and identity.
SAML 2.0 Workflow
A simple SAML workflow inorder to understand the process better can be shown as below:
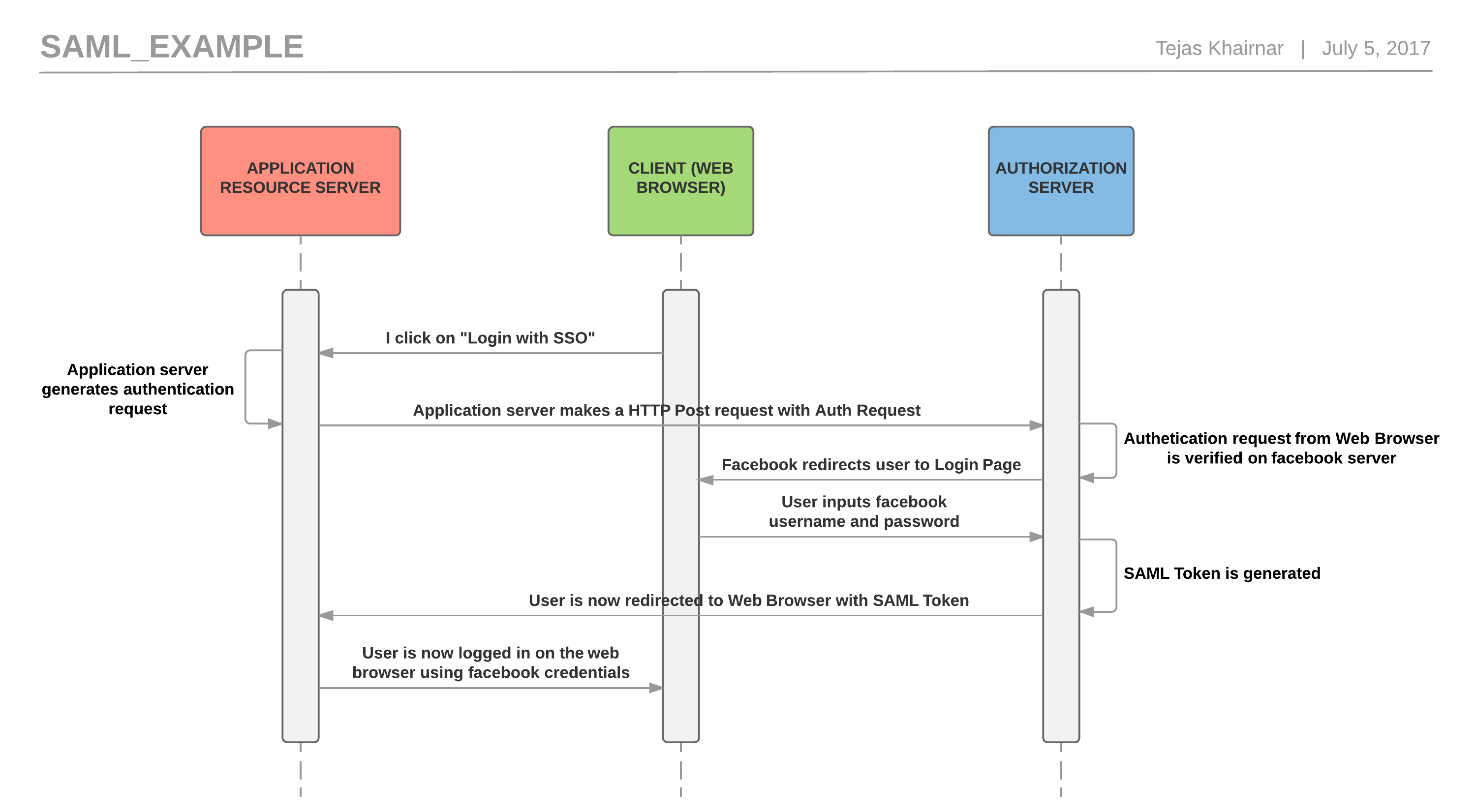
The above example can be described in words as:
- First I click on “login with SSO”. This is similar to clicking on login with Facebook on your web browser.
- Then the application server which receives the login request generates the authentication request to be sent to the Authorization server.
- Now the application server makes an HTTP Post request with an Authentication request to the authorization server.
- This authentication request is then verified on the authorization server.
- Now once verified, the authorization server redirects the user’s browser session on its login page.
- This is where the user inserts his/her username/password for facebook (for example) on the login page.
- Now if the credentials for that user are verified on authorization server (for example Facebook side), then and only then the authorization server at Facebook will generate a SAML token.
- The user is now redirected to the web application with the SAML Token.
- Using this SAML token the application authenticates the user using Facebook credentials and still does not store user’s username/password.
Limitations about SAML 2.0
There are certain cases where SAML specification does not work or to be specific I should say difficult to make it work. One case is for Thick Client or Native applications.
Because in this case, Step 8, is difficult to achieve. In the above example Step 8 is achieved by using HTTP Redirect binding. This makes the user redirected back to the mentioned URL. However, this cannot be achieved when we are dealing with a native application installed on your machine or a mobile application installed on your phone. One harder way in order to get this working is to scrape the SAML token from the HTTP request coming from the authorization server using a proxy server in between. Once you pull out the SAML token you can make an HTTP request using this token in order to get access.
OAuth Basics
Unlike SAML 2.0, OAuth 2.0 is a protocol for authorization. It focuses on how to deliver authorization flows for web applications, desktop applications, mobile phones and other devices. In OAuth there are following main actors:
- Service Provider (Resource Server): This is the web server you are trying to access information on.
- Client: It can be the browser based web application, mobile application, desktop application or even a server-side application.
- Identity Provider (Authorization Server): This is the server that owns the user identities and credentials. It’s who the user actually authenticates and authorizes with.
OAuth Workflow
A simple OAuth workflow in order to understand the process better can be shown as below:
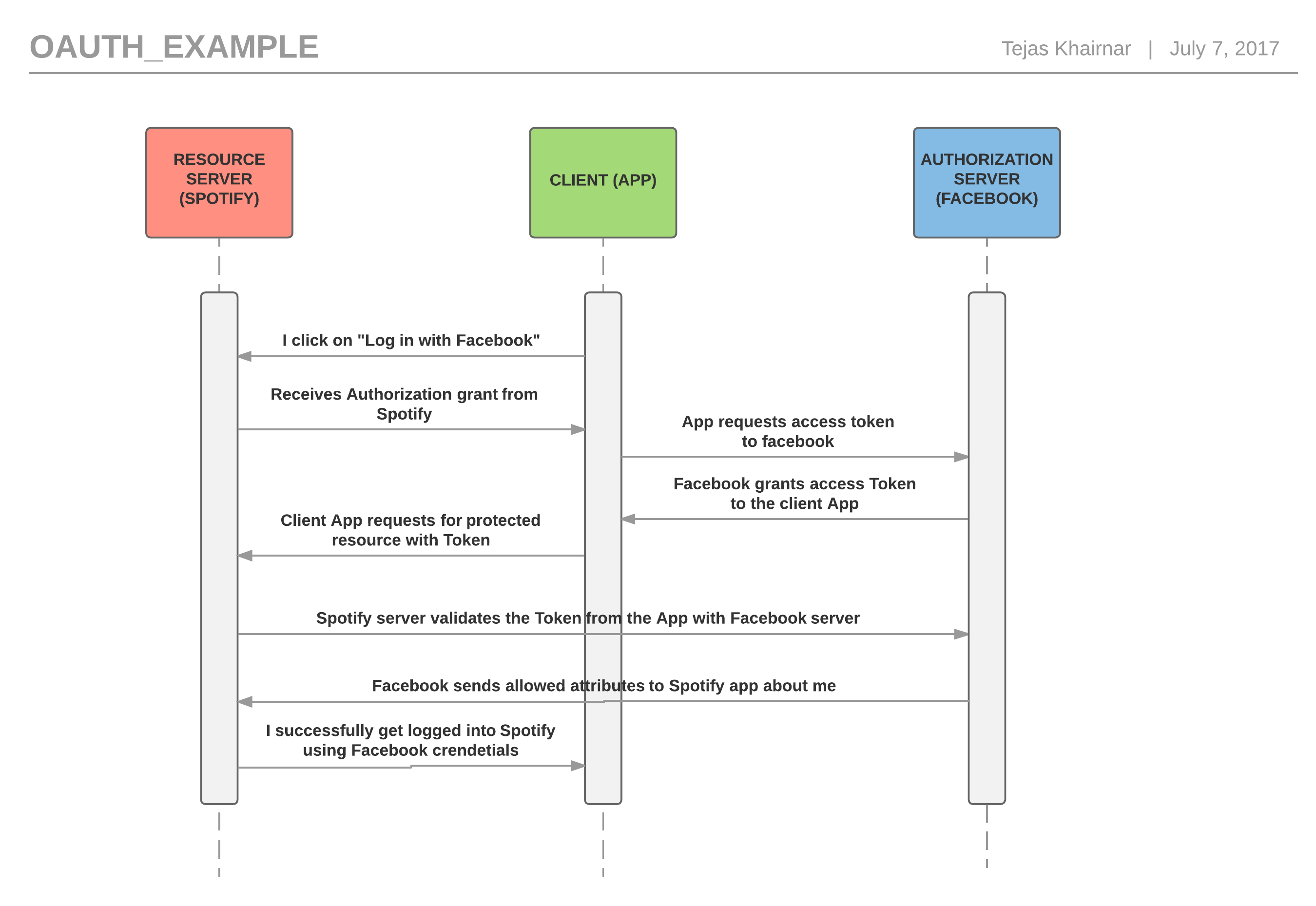
- First I click on “login with Facebook” on Spotify mobile app.
- The Service Provider at Spotify gives me an authorization grant in order to login with Facebook.
- Now, my mobile app will use this authorization grant in order to request an access token from the Identity Provider, in our example, Facebook.
- Facebook checks whether the authorization grant is valid and grants the access token if valid. This access token is now used by the client (in our case Spotify mobile app)
- Now, when Spotify (Service Provider) receives this access token, it might not directly let you access the app as logged in user. It will validate this access token again with Facebook. If Facebook finds this token valid, then and only then it will send back the user information to Spotify.
- After successful validation of access token, Spotify logs in the user with his/her Facebook credentials without owning the user’s username/password.
This is the most common OAuth flow. There are various other things about OAuth such as refresh tokens, where the client asks for refresh tokens to the Authorization server instead of again authenticating itself via the common OAuth flow, but I am keeping this post an introductory to Single-Sign On process.
The difference between SAML 2.0 and OAuth 2.0 here is, OAuth does not assume that the client is a web browser. And also in the OAuth flow, the token is sent in the HTTP redirects as the query parameter. Hence it is easy to absorb on the application side too. Other difference is SAML 2.0 has the identity information in the SAML token itself, while OAuth token does not have that. It again has to validate the token against the Identity provider which increases the request/response overhead but is more SECURE.
This is it for now on SAML & OAuth. Now that we are on this topic would just like to tell you there is something more called “OpenID Connect” similar to SAML & OAuth. I will leave you here to search about it.
References:
- The OAuth 2.0 Authorization Framework
- Security Assertion Markup Language (SAML) 2.0 Profile for OAuth 2.0 Client Authentication and Authorization Grants
- Choosing an SSO Strategy: SAML vs OAuth2
- Dev Overview of SAML
18 Jun 2017
Every company has some kind of internet access policy in order to keep their network secure. According to this policy sometimes you cannot access social media websites like facebook, instagram etc. In this blog post I will guide you on how to ssh into your home computer and access blocked websites at your workplace leveraging the same. ** Violation of Company’s security policy can cost you **
Installing ssh on your home machine
For Mac Users:
Its pretty straight forward. Go to “System Preferences” > “Sharing” > “Remote login”. Enable remote login for particular usrers on the Mac. Some of the configurations related to securing ssh are explained later in this post.
For Linux Users:
Follow the following commands in order to install OpenSSH-Server on your linux box.
sudo apt-get install openssh-server
Just follow the instructions and OpenSSH-server will be installed on your linux box.
Securing SSH service
Now, we also have to secure the ssh service we just started on our machine. All the configuration related to ssh service are in the file “sshd_config”. Any changes made in this file are the changes made for the ssh service.
You should make the following changes to implement some level of secuirty for your ssh service. (These changes are mostly generic for ssh service keeping aside the platform)
Use public/private key pairs for authentication instead of passwords
Generate a passphrase-protected SSH key for every computer that need access to the ssh home machine. In our case we will create this on our work machine.
ssh-keygen -t rsa -b 2048 -v
Here ssh-keygen unix based utility generates authentication keys for ssh. Using -t option you can specify the type of key to create. I am specifying RSA as cryptography type for my ssh-key because RSA algorithm is right now compatible with most of the ssh versions and -b option to specify the number of bits to be generated. 2048 bit RSA key is pretty strong against factoring of RSA modulus. I use -v to get pretty messages about the key generation. Btw, its verbose.
ssh-copy-id -i ~/.ssh/id_rsa.pub user@publicip
Here we copy the key we generated for the work machine into the home machine. The user will be the users who are allowed access to your home machine. The “publicip” thing I will exaplin further.
Disable Password Access
Now that we have configured keys in order to ssh into home machine we can disable the password based access. This prevents us from the password bruteforce attacks.
The password access can be disabled by:
#PasswordAuthentication yes
PasswordAuthentication no
Disable RootLogin
We should not allow root users to login via ssh. This can be done by:
#PermitRootLogin no
PermitRootLogin no
Limit the number of users that can ssh into your home machine
We can achieve this by adding a line into sshd_config file about allowing specific users or specific group of users to login via ssh.
This can be done by adding this line at the end of sshd_config:
AllowUsers user1 user2
Using ssh protocol 2
SSh protocol 1 has number of security vulnerabilities of which exploits are available publicly. Hence we should only use ssh protocol 2 for our purpose.
This can be done by uncommenting the protocol line in sshd_config and mentioning the number ‘2’.
Knowning your public IP address
Now that we have our SSH service running on our home machine we can move ahead on how to access your home machine from work place. The first and foremost requirement in order to achieve this is to know the Public IP of your home network. I am assuming that you have a router placed at your home to which all your devices are connected. There are many ways in order to find the Public IP of your router. The following are two of my ways:
Configuring your router
We know the Public IP of our router now. Lets go ahead with some network related stuff to make this work.
Public IP’s are accessible from everywhere in the world. That’s why they are called Public. :)
But your home machine is NATted behind your router. By this I mean that your router performs some kind of address translation from PrivateIP > PublicIP and vice versa whenever you access internet at home. You can verfiy this by checking your IP address configuration. It would be most probably start with “192.168”. Now, the question is, how will our router recognize that I want to access which device on myhome network?
There are many ways to achieve this, but the first thought ways are as:
Port Forwarding
“A port forward is a way of making a computer on your home or business network accessible to computers on the internet, even though they are behind a router. (https://portforward.com/)”
In order to make this work we have to login into the management console of the home router and make the configuration.
For me the configuration tab was in the advanced networking mode.
Here you need to add:
"Service Name" = ssh(22)
"Action" = Allow
"LAN IP Address" = IP address of your home machine
"WAN IP Address" = Any (You can spcify the public IP of y our office network to be more secure)
DMZ
Demilitiarized Zone is a physical of logical sub-network that separates an internal local area network(LAN) from other untrusted networks, usually the Internet[http://searchsecurity.techtarget.com/definition/DMZ]. This means that the machines/servers in the DMZ are accessible from the outside network. To achieve our goal, instead of port forwarding, we can make you machine sit in the DMZ so that it will be publicly accessible. BUT, there is a big but here, as you might have guessed making your machine publicly avaible doesn’t sound much secure. You are right. It would not be secure. Hence, let’s stop this discussion here.
But if anyone want to configure there machine to reside in the DMZ, you can do so by sepcifyinh your private IP into the router DMZ tab. After that the router will take care of it. (Again, I am assuming that your router supports DMZ setup)
Dynamic DNS
While performing port forwarding configuration when I say “IP address of your machine”, you might question that my router leases IP using DHCP. Then how can I gurantee that every time I will have the same private IP adress? True. This can be a problem all the time. But, thanks to Dynamic DNS service. Dynamic DNS is a method of autmatically updating a name server in the DNS. So basically the only thing you have to understand is that it will provide you with a constant domain name for your local machine even though it will have different IP’s. This can be configured on your router once you have the name configured using services like noip.
SSH Reverse tunneling
In order to achieve the goal of bypassing corporate firewall, we use the technique of SSH Reverse Tunneling. Generally corporate firewalls are desgined to block any incoming connections from outside network. Also, for security purposes many social media websites are blocked on the firewall. But, many times a connection going from inside to outside is not blocked. Otherwise, it will be difficult to work. Right?
Hence, we leverage this to our advantage and in this technique we try to open a connection from inside to outside.
In order to make a SSH reverse tunnel to your home PC use the below command:
ssh -R 4444:localhost:22 user@publicip
The -R option in above command will forward the give port on remote server to the given host and port on local side. In the above command, we use 4444 as the port on our home machine to connect back to our work machine. “user@publicip” means you are connecting to your home machine.
Now, from your home machine you have to connect back to the office machine using the tunnel established. This time you will be able to connect because a legitimate port is open through the corporate firewall from inside to outside.
ssh -p 4444 user@localhost
This way you are connecting back to the work machine through the tunnel.
Now that the tunnel is established, in order to browse the blocked website we have to setup something called as SOCKS proxy on our browser.
In order to do so,
open "Mozilla-firefox"
go-to "preferences"
click "advanced"
"Network" > "Settings" > "SOCKS Host" > localhost: "Port" > 4444
Now all the traffice from this browser will get tunnel through the SSH reverse tunnel we established and will go via your home internet.
This will enable us to access all the blocked websites with ease.
SSH reverse tunneling technique is highly monitored in coportate networks. Your coporate IT team might not like it. Please think twice before performing this. I have written this blog post only to learn more about this technique. Hope this helps. :) :)